Megalaga 8 - Spam Protection
NOTE:
This tutorial is most likely not compatible with versions of SGDK above 1.70.
Unfortunately, I simply do not have the capacity or ability to update them right now. Read more about it here. Sorry.
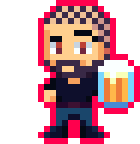
Last time I mentioned that there is a potential issue concerning our game which was related to the bullet pool. And now I’m going to reveal the secret (and of course fix the issue)!
The issue stems from the fact that both the player and all enemies draw from the same bullet pool. The player isn’t actually limited in how many bullets they can fire; before a bullet is fired, we only check whether bulletsOnScreen < MAX_BULLETS
is true. This means that if the player keeps firing continuously, there will almost never be a free bullet for an enemy to shoot, because the player is using all bullets available in the pool!
This of course makes the game super easy, which is not what we want. I mean, if we wanted an easy game, we wouldn’t have let the enemies shoot in the first place, right? So let’s fix that.
Start off with a new define and a new variable near the top of main.c
:
#define MAX_PLAYER_BULLETS 3
u16 shotByPlayer = 0;
The variable shotByPlayer
will keep track of how many of the currently active bullets have been shot by the player. We will compare this value to MAX_PLAYER_BULLETS
whenever the player tries to fire. This way some of the bullets in the pool will be reserved for the enemies.
Let’s move on to shootBullet()
now to implement this! You’ll see that first of all, we check whether a bullet has been fired by the player and store that result in fromPlayer
. Then we check whether we have reached the maximum amount of bullets allowed on screen. We want to leave both of these checks unchanged, but we will add a new one right at the start of the if (bulletsOnScreen < MAX_BULLETS)
body:
if(fromPlayer == TRUE){
if(shotByPlayer >= MAX_PLAYER_BULLETS){
return;
}
}
So now the player can only fire if a) there is still room on screen for another bullet and b) if MAX_PLAYER_BULLETS
hasn’t been reached yet. If the player has fired all of their allotted bullets, we simply return
out of shootBullet()
without firing anything. The player will then have to wait until one of the bullets they have fired hits an enemy or goes offscreen.
But for this to work we need to keep shotByPlayer
updated. Let’s start doing this in shootBullet()
while we’re still here!
After we use reviveEntity
to revive the bullet we want to fire, we check whether the bullet is going to be fired by the player to set the velocity accordingly. Looks like the perfect spot to increase our counter! So add a line to make the statement look like this:
if(fromPlayer == TRUE){
b->vely = -3;
shotByPlayer++;
} else{ ... }
This way shotByPlayer
will only get incremented if the player shoots a bullet, not an enemy. But of course we’ll also have to decrease the value somewhere! We’ll do this twice. The first time is in positionBullets()
. Inside of the if-statement where we check whether the current bullet has left the top of the screen, add a line to make it look like this:
if (b->y + b->h < 0)
{
killEntity(b);
bulletsOnScreen--;
shotByPlayer--;
}
This will free up a bullet in the pool when the player misses an enemy. And now we of course have to decrease the value when the player doesn’t miss! This will happen inside of handleCollisions()
.
Inside the innermost if-statement body of that function (so inside of if (collideEntities(b, e)){}
), add the line so it looks like this:
//...
enemiesLeft--;
bulletsOnScreen--;
shotByPlayer--;
//...
And there we go, that’s all we need to do! Now we keep track of how many bullets the player has fired and prevent them from firing more once the limit has been reached. This way there is no way to cheese the game by spamming bullets and keeping the pool empty for the enemies. You can of course decrease MAX_PLAYER_BULLETS
to make the game tougher on players if you want!
Our game is even more polished now, but one thing is still missing: Sound! It wouldn’t be a space shooter if you didn’t hear the pew pew of lasers and the krakoom of exploding enemies. So next time we’ll talk about sound drivers and how to make our game less quiet! Until then, be excellent to each other!
If you've got problems or questions, join the official SGDK Discord! It's full of people a lot smarter and skilled than me. Of course you're also welcome to just hang out and have fun!
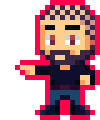
Want To Buy Me a Coffee?
Coffee rules, and it keeps me going! I'll take beer too, though.
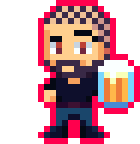
Check out the rest of this tutorial series!
Comments
By using the Disqus service you confirm that you have read and agreed to the privacy policy.
comments powered by Disqus