Drawing Tiles From Code
NOTE:
This tutorial is most likely not compatible with versions of SGDK above 1.70.
Unfortunately, I simply do not have the capacity or ability to update them right now. Read more about it here. Sorry.
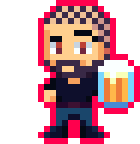
So far, when we wanted to have tiles in our games, we always created them in a graphics program and imported them (incidentally, I have a list of 4 programs you can use to create Mega Drive graphics). And I mean, that makes sense, right? If you need graphics then you draw graphics. And since SGDK can import and process regular .png files, that really is the best and most logical way to get tile graphics into your game.
But did you know that you can also skip a step and draw tiles directly from code? Yep, that’s a possibility! Let’s look at how you’d go about doing that.
First off, generate a new project (you can find out how in my Megapong tutorial). Then just open main.c
in your code editor. We won’t be bothering with any assets this time around, so you can ignore the res
folder.
Alright, let’s code ourselves a tile! Within main()
, create an array of type u32
:
const u32 tile[8] =
{
0x00000000,
0x00000000,
0x00000000,
0x00000000,
0x00000000,
0x00000000,
0x00000000,
0x00000000
};
And that’s our tile! Don’t worry, I’ll explain in a second. First we’ll load and draw our tile to the screen with the following two lines of code:
VDP_loadTileData(tile, 1, 1, 0);
VDP_setTileMapXY(BG_A, 1, 5, 5);
This will load our array as a tile at index 1
and draw it at tile-position 5,5
. Now compile the game and you’ll see…nothing! Nothing at all. That’s because our tile is just a solid 8x8 pixel chunk of black. Let me explain how to change that and actually draw something!
Alright, take a look at the array. You’ll notice that we have 8 rows of values, and each row consists of 8 digits (ignoring the hex prefix of 0x
). 8x8, huh? Exactly like a Mega Drive tile!
Basically, the array as it’s formatted above is nothing but a canvas. Each digit corresponds to a pixel in the 8x8 canvas of the tile. Each digit specifies what color its pixel should be, with the value being the index of the desired color in the current color palette. Each palette on the Mega Drive can store 16 colors, meaning each digit of the array could be anything from 0 to F (remember, these are hex values).
But enough theory, let me show you some examples. First let’s add some colors to our palette (through code, naturally) at the beginning of main()
, before we define and load our tile:
VDP_setPaletteColor(1, RGB24_TO_VDPCOLOR(0xFF0000)); //Red
VDP_setPaletteColor(2, RGB24_TO_VDPCOLOR(0x00FF00)); //Green
VDP_setPaletteColor(3, RGB24_TO_VDPCOLOR(0x0000FF)); //Blue
VDP_setPaletteColor(4, RGB24_TO_VDPCOLOR(0xFFFF00)); //Yellow
This will set the first 4 colors in the first palette (PAL0
) to be red, green, blue and yellow respectively.
Okay, now change our array to look like this:
const u32 tile[8] =
{
0x11111111,
0x10000001,
0x10000001,
0x10000001,
0x10000001,
0x10000001,
0x10000001,
0x11111111
};
Now the outer edges of the array consists of 1
s, which point to the color at index 1 of the palette…which in our case is red. So compile the game, and…
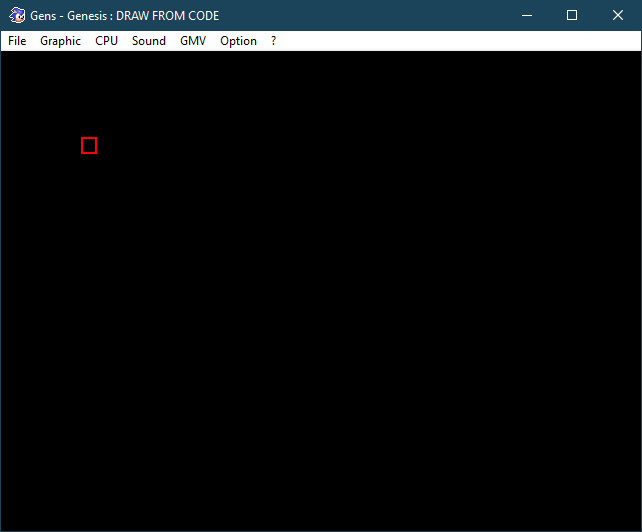
…success! We have successfully drawn a red square just from code. Who needs artists?
Now if you wanted to for example add a yellow block in middle of the tile, you’d do it like this:
const u32 tile[8] =
{
0x11111111,
0x10000001,
0x10000001,
0x10044001,
0x10044001,
0x10000001,
0x10000001,
0x11111111
};
This should add four pixels of color 4 (= yellow) to the center of the tile. After a quick compile we’ll see that this is indeed the case!
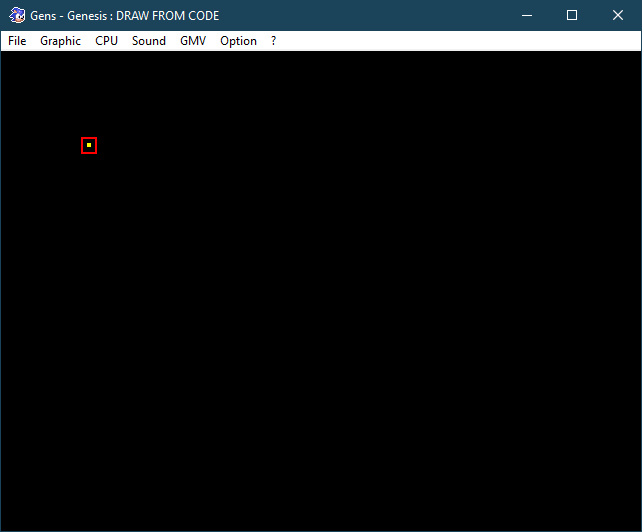
The array is simply the canvas on which you draw, but instead of using the mouse to place colored pixels on an image, you use the keyboard to place color index values in an array. And while the latter approach is of course more cumbersome, it also sounds pretty hacker, wouldn’t you say?
If you've got problems or questions, join the official SGDK Discord! It's full of people a lot smarter and skilled than me. Of course you're also welcome to just hang out and have fun!
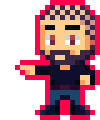
Want To Buy Me a Coffee?
Coffee rules, and it keeps me going! I'll take beer too, though.
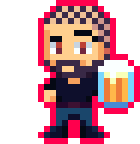
Check out the rest of this tutorial series!
Comments
By using the Disqus service you confirm that you have read and agreed to the privacy policy.
comments powered by Disqus